自定义异常页面
从 SpringBoot 错误机制源码分析,知道当遇到错误情况时候,
- SpringBoot 会首先返回到模版引擎文件夹下的
/error/HTTP
状态码 文件,
- 如果不存在,则检查去模版引擎下的
/error/4xx
或者 /error/5xx
文件,
- 如果还不存在,则检查静态资源文件夹下对应的上述文件。
并且在返回时会共享一些错误信息,这些错误信息可以在模版引擎中直接使用。
1 2 3 4 5 6 7 8
| errorAttributes.put("timestamp", new Date()); errorAttributes.put("status", status); errorAttributes.put("error", HttpStatus.valueOf(status).getReasonPhrase()); errorAttributes.put("errors", result.getAllErrors()); errorAttributes.put("exception", error.getClass().getName()); errorAttributes.put("message", error.getMessage()); errorAttributes.put("trace", stackTrace.toString()); errorAttributes.put("path", path);
|
在 templates/error 下自定义 404.html 页面:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!doctype html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>[[${status}]]</title> <link href="/webjars/bootstrap/4.1.3/css/bootstrap.min.css" rel="stylesheet"> </head> <body > <div class="m-5" > <p>错误码:[[${status}]]</p> <p>信息:[[${message}]]</p> <p>时间:[[${#dates.format(timestamp,'yyyy-MM-dd hh:mm:ss ')}]]</p> <p>请求路径:[[${path}]]</p> </div>
</body> </html>
|
随意访问不存在路径得到:
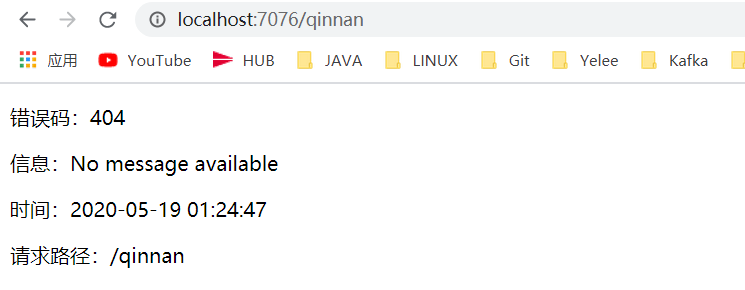
自定义错误JSON
根据上面的 SpringBoot 错误处理原理分析,得知最终返回的 JSON 信息是从一个 map 对象中转换出来的,那么,只要能自定义 map 中的值,就可以自定义错误信息的 json 格式了。直接重写 DefaultErrorAttributes
类的 getErrorAttributes
方法即可。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| package com.qn.config;
import org.springframework.boot.web.servlet.error.DefaultErrorAttributes; import org.springframework.stereotype.Component; import org.springframework.web.context.request.WebRequest; import java.util.HashMap; import java.util.Map;
@Component public class ErrorAttributesCustom extends DefaultErrorAttributes {
@Override public Map<String, Object> getErrorAttributes(WebRequest webRequest, boolean includeStackTrace) { Map<String, Object> map = super.getErrorAttributes(webRequest, includeStackTrace); String code = map.get("status").toString(); String message = map.get("templates/error").toString(); HashMap<String, Object> hashMap = new HashMap<>(); hashMap.put("code", code); hashMap.put("message", message); return hashMap; } }
|

全局异常处理
使用 @ControllerAdvice
结合 @ExceptionHandler
注解可以实现统一的异常处理,@ExceptionHandler
注解的类会自动应用在每一个被 @RequestMapping
注解的方法。当程序中出现异常时会层层上抛
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| package com.qn.handler;
import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseBody;
@ControllerAdvice public class ExceptionHandle { @ResponseBody @ExceptionHandler(Exception.class) public String handleException(Exception e) { return e.getMessage(); } }
|